Recommanded Books |
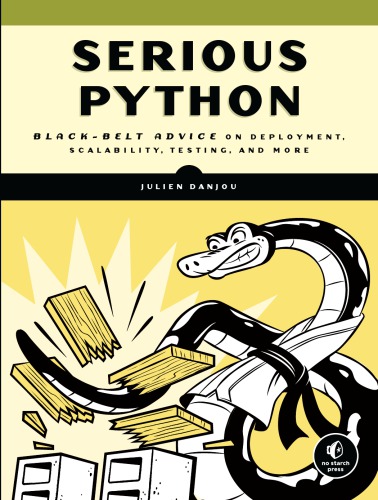 | Title | Serious Python: Black-Belt Advice on Deployment, Scalability, Testing, and More |
ISBN | 978-1-593-27878-6 |
Author | Julien Danjou |
Year | 2019 |
Publisher | No Starch Press |
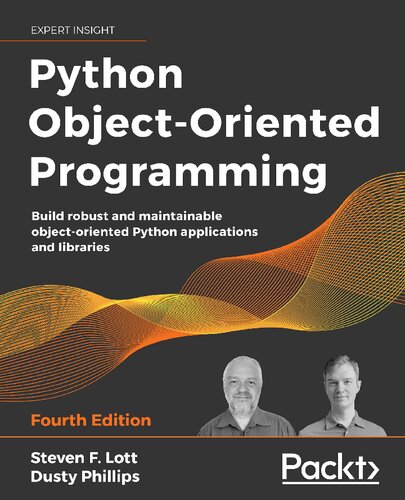 | Title | Python Object-Oriented Programming: Build robust and maintainable object-oriented Python applications and libraries |
ISBN | 978-1-801-07726-2 |
Author | Steven F. Lott, Dusty Phillips |
Year | 2021 |
Publisher | Packt Publishing |
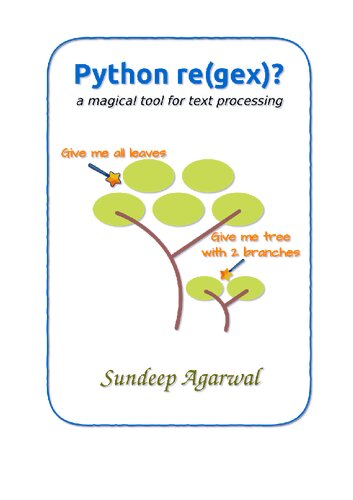 | Title | Python re: a magical tool for text processing |
ISBN | |
Author | Sundeep Agarwal |
Year | 2019 |
Publisher | Sundeep Agarwal |
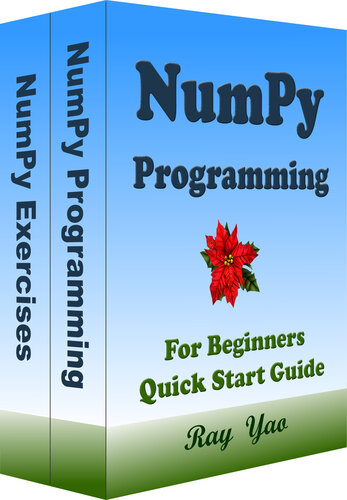 | Title | Numpy Programming, For Beginners, Quick Start Guide: Numpy Language Crash Course Tutorial & Exercises |
ISBN | B09446DZQH |
Author | Yao, Ray |
Year | 2021 |
Publisher | In Easy Step By Step, Teach Yourself eBook & Book |
.jpg) | Title | Python Data Analytics: Mastering Python for Effective Data Analysis and Visualization |
ISBN | B0CW28VQJ4 |
Author | Floyd Bax |
Year | 2024 |
Publisher | FLOYD BAX; 1st edition |
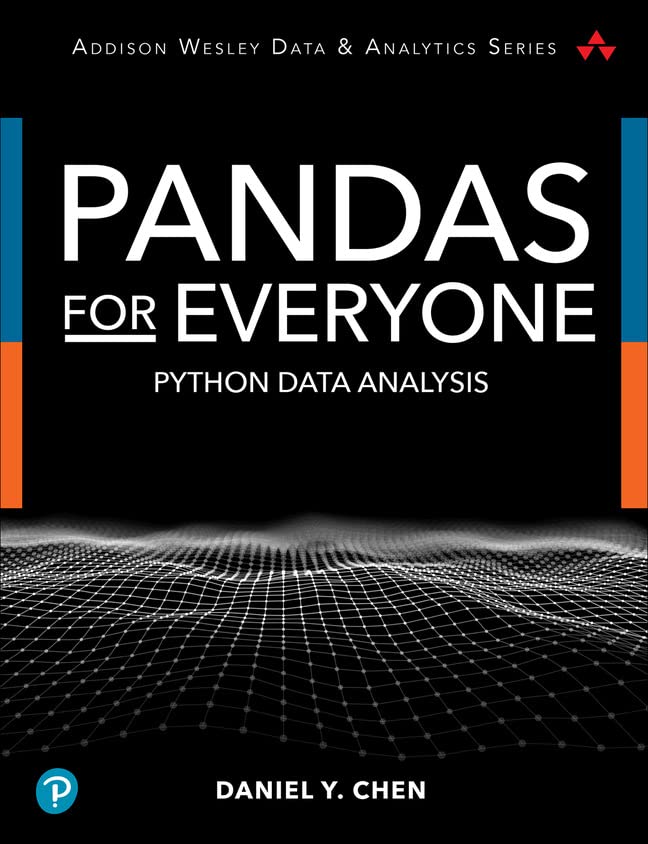 | Title | Pandas for Everyone: Python Data Analysis |
ISBN | 978-0-137-89115-3 |
Author | Daniel Chen |
Year | 2023 |
Publisher | Addison-Wesley Professional |
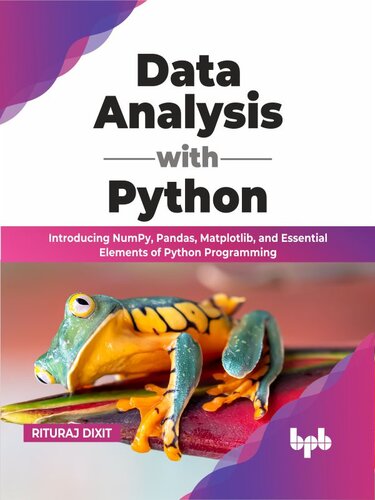 | Title | Data Analysis with Python: Introducing NumPy, Pandas, Matplotlib, and Essential Elements of Python Programming |
ISBN | 978-9-355-51065-5 |
Author | Rituraj Dixit |
Year | 2023 |
Publisher | BPB Publications |
.jpg) | Title | Python for Data Analysis: Data Wrangling with pandas, NumPy, and Jupyter |
ISBN | 978-1-098-10403-0 |
Author | Wes McKinney |
Year | 2022 |
Publisher | O'Reilly Media |
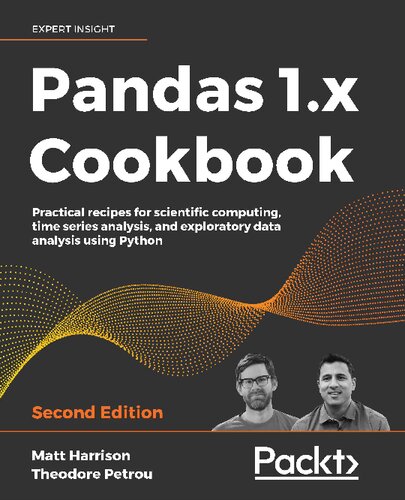 | Title | Pandas 1.x Cookbook: Practical recipes for scientific computing, time series analysis, and exploratory data analysis using Python |
ISBN | 978-1-839-21310-6 |
Author | Matt Harrison, Theodore Petrou |
Year | 2020 |
Publisher | Packt Publishing |
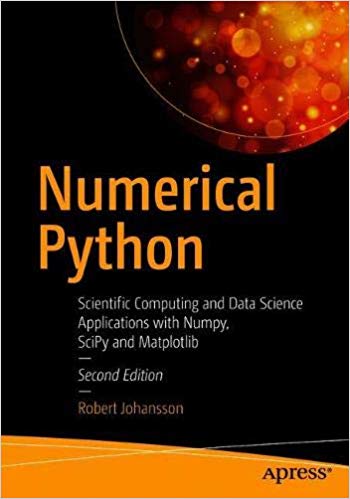 | Title | Numerical Python: Scientific Computing and Data Science Applications with Numpy, SciPy and Matplotlib |
ISBN | 978-1-484-24245-2 |
Author | Robert Johansson |
Year | 2019 |
Publisher | Apress |
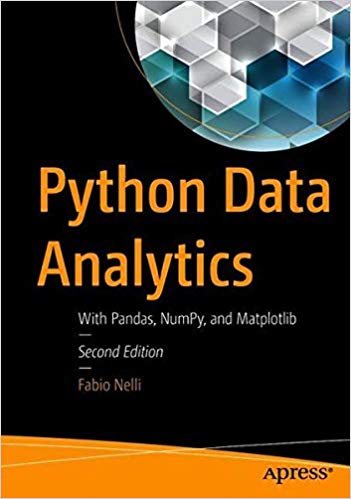 | Title | Python Data Analytics: With Pandas, NumPy, and Matplotlib |
ISBN | 978-1-484-23912-4 |
Author | Fabio Nelli |
Year | 2018 |
Publisher | Apress |
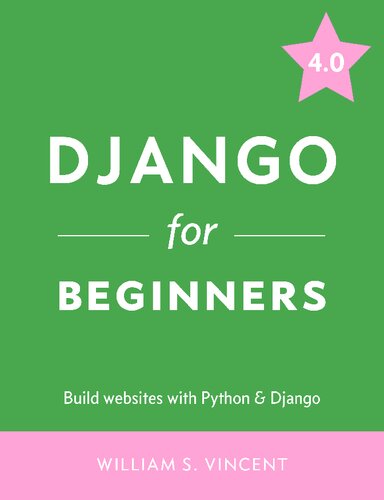 | Title | Django for Beginners: Build websites with Python & Django |
ISBN | |
Author | William S. Vincent |
Year | 2022 |
Publisher | Leanpub |
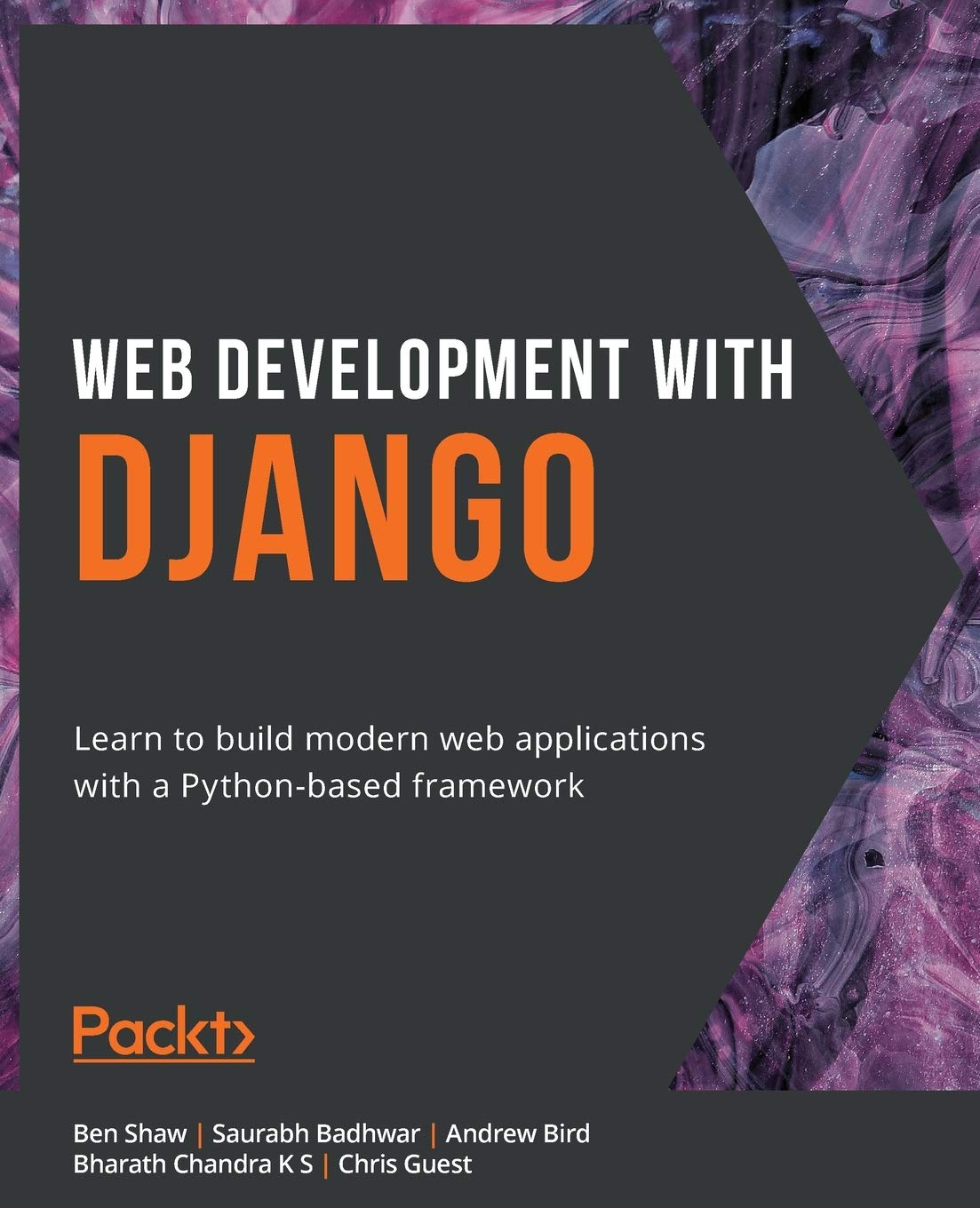 | Title | Web Development with Django: Learn to build modern web applications with a Python-based framework |
ISBN | 978-1-839-21250-5 |
Author | Ben Shaw, Saurabh Badhwar, Andrew Bird, Bharath Chandra K S, Chris Guest |
Year | 2021 |
Publisher | Packt Publishing |
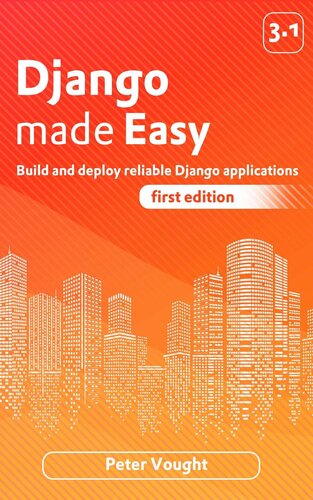 | Title | Django Made Easy: Build and deploy reliable Django applications |
ISBN | B08RXZ6JPP |
Author | Vought, Peter |
Year | 2021 |
Publisher | |
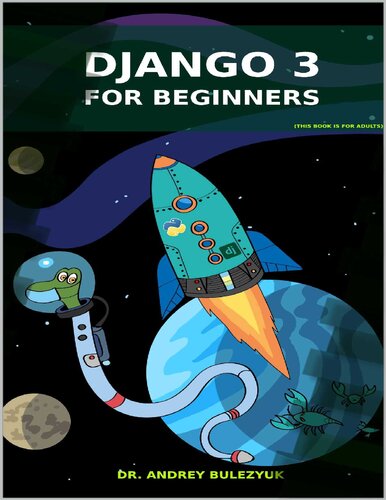 | Title | Django 3: For Beginners |
ISBN | 979-8-571-46247-1 |
Author | Andrey Bulezyuk |
Year | 2021 |
Publisher | |
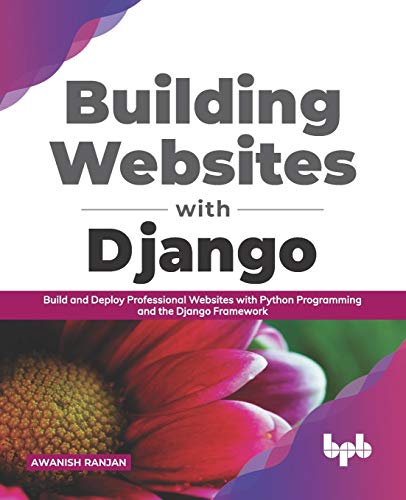 | Title | Building Websites with Django: Build and deploy professional websites with Python programming and the Django framework |
ISBN | 978-9-389-32828-8 |
Author | Awanish Ranjan |
Year | 2021 |
Publisher | BPB Publications |
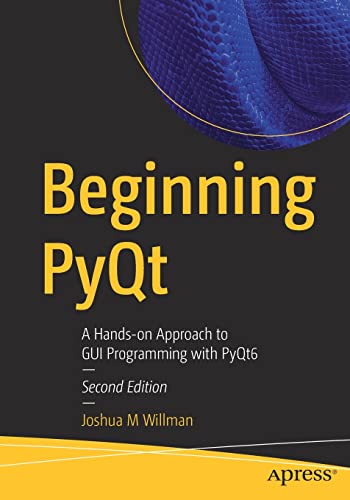 | Title | Beginning PyQt: A Hands-on Approach to GUI Programming with PyQt6 |
ISBN | 978-1-484-27998-4 |
Author | Joshua M Willman |
Year | 2022 |
Publisher | Apress |
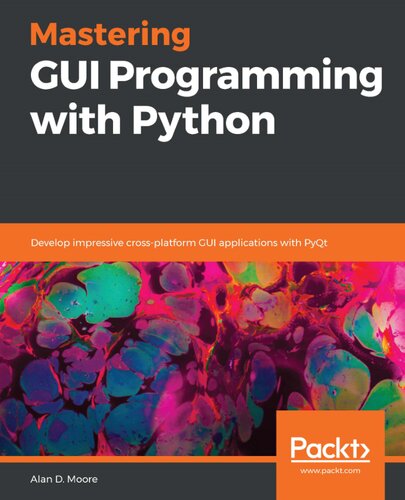 | Title | Mastering GUI Programming with Python: Develop impressive cross-platform GUI applications with PyQt |
ISBN | 978-1-789-61290-5 |
Author | Alan D. Moore |
Year | 2019 |
Publisher | Packt Publishing |
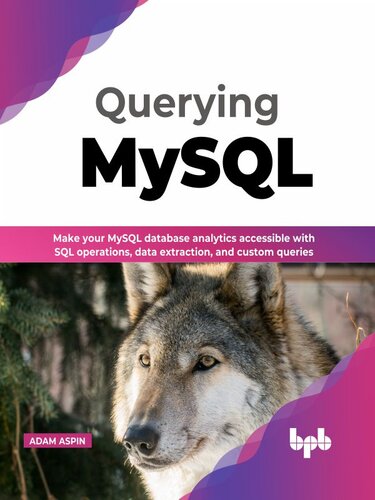 | Title | Querying MySQL |
ISBN | 978-9-355-51267-3 |
Author | Aspin, Adam |
Year | 2022 |
Publisher | BPB Publications |
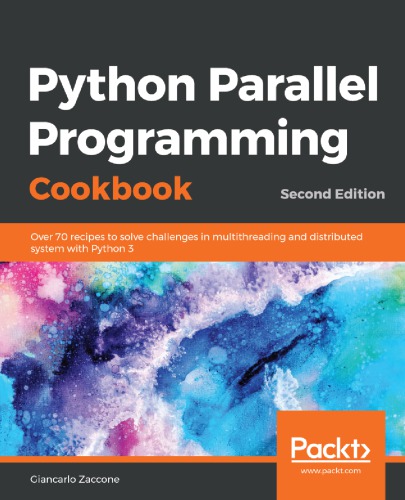 | Title | Python Parallel Programming Cookbook: Over 70 recipes to solve challenges in multithreading and distributed system with Python 3 |
ISBN | 978-1-789-53373-6 |
Author | Giancarlo Zaccone |
Year | 2019 |
Publisher | Packt Publishing |
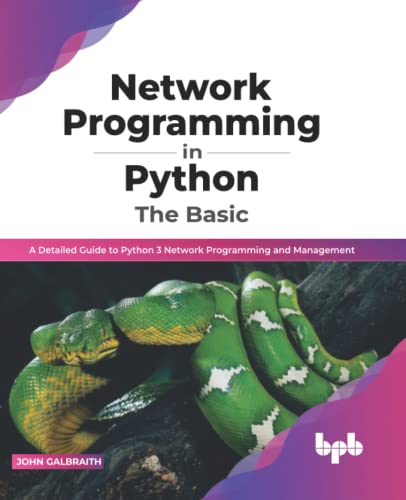 | Title | Network Programming in Python: The Basic: A Detailed Guide to Python 3 Network Programming and Management |
ISBN | 978-9-355-51257-4 |
Author | John Galbraith |
Year | 2022 |
Publisher | BPB Publications |