Recommanded Books |
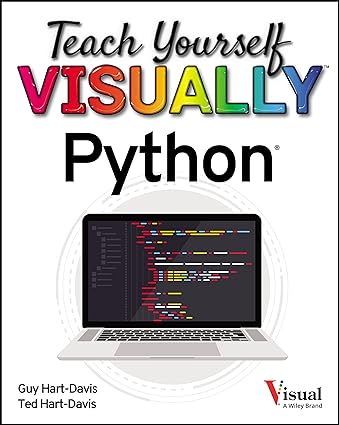 | Title | Teach Yourself Visually Python |
ISBN | 978-1-119-86025-9 |
Author | Guy Hart-Davis, Ted Hart-Davis |
Year | 2022 |
Publisher | Wiley |
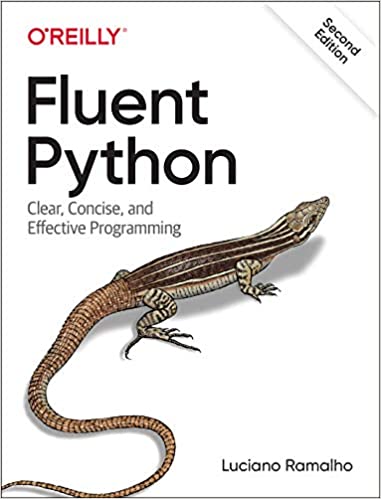 | Title | Fluent Python: Clear, Concise, and Effective Programming |
ISBN | 978-1-492-05635-5 |
Author | Luciano Ramalho |
Year | 2021 |
Publisher | O'Reilly Media, Inc. |
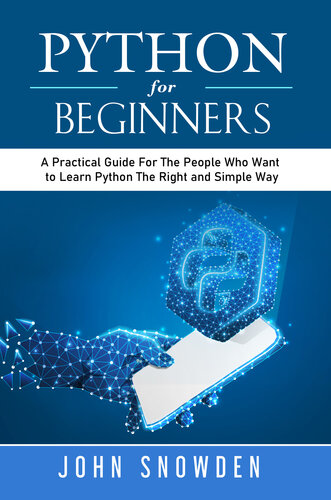 | Title | Python For Beginners: A Practical Guide For The People Who Want to Learn Python The Right and Simple Way (Computer Programming Book 1) |
ISBN | 979-8-575-56608-3 |
Author | Snowden, John |
Year | 2021 |
Publisher | |
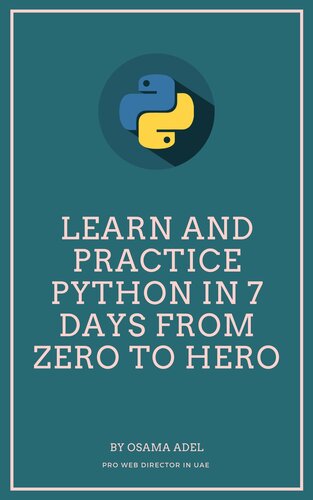 | Title | Learn and Practice Python in 7 Days From Zero To Hero: Learn Python Step by Step,Learn Python in 7 days |
ISBN | B08SJ2QL8J |
Author | Adel, Osama |
Year | 2021 |
Publisher | |
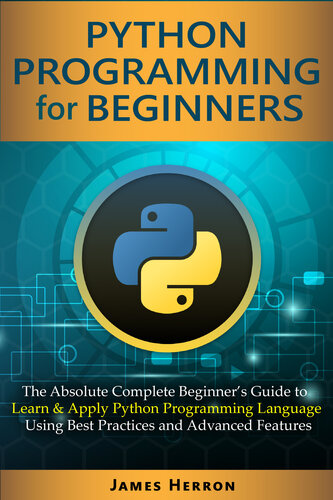 | Title | Python Programming For Beginners: The Absolute Complete Beginner’s Guide to Learn and Apply Python Programming Language Using Best Practices and Advanced Features |
ISBN | 979-8-595-00629-3 |
Author | Herron, James |
Year | 2021 |
Publisher | Independently published |
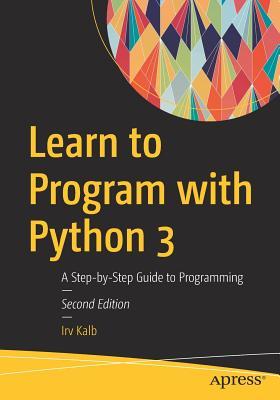 | Title | Learn to Program with Python 3 |
ISBN | 978-1-484-23878-3 |
Author | Irv Kalb |
Year | 2018 |
Publisher | Apress |
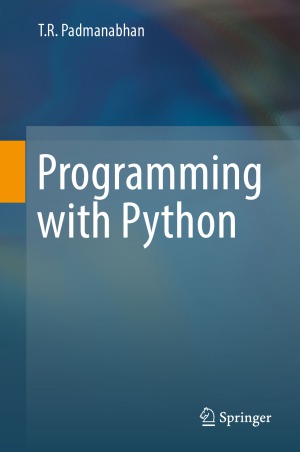 | Title | Programming with Python |
ISBN | 978-9-811-03276-9 |
Author | T.R. Padmanabhan |
Year | 2017 |
Publisher | Springer |
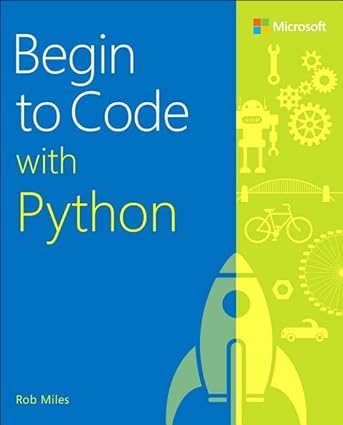 | Title | Begin to code with Python |
ISBN | 978-1-5093-0452-3 |
Author | Rob Miles |
Year | 2018 |
Publisher | Microsoft |
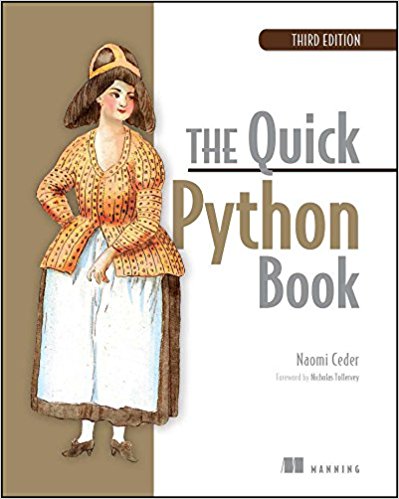 | Title | The Quick Python Book |
ISBN | 978-1-617-29403-7 |
Author | Naomi Ceder |
Year | 2018 |
Publisher | Manning Publications |
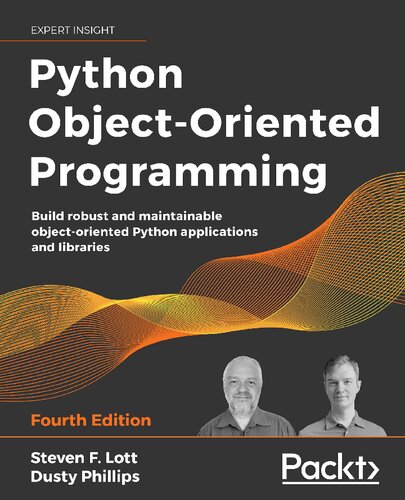 | Title | Python Object-Oriented Programming: Build robust and maintainable object-oriented Python applications and libraries |
ISBN | 978-1-801-07726-2 |
Author | Steven F. Lott, Dusty Phillips |
Year | 2021 |
Publisher | Packt Publishing |
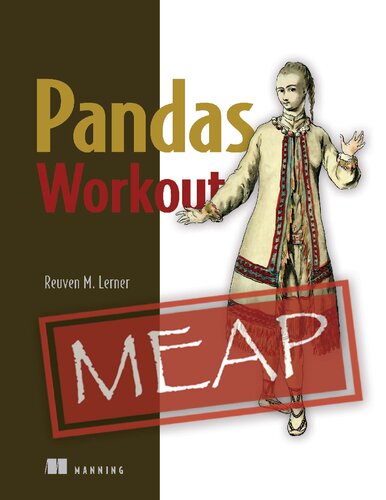 | Title | Pandas Workout Version 6 |
ISBN | 978-1-617-29972-8 |
Author | Reuven M. Lerner |
Year | 2022 |
Publisher | Manning Publications |
.jpg) | Title | Python Data Analytics: Mastering Python for Effective Data Analysis and Visualization |
ISBN | B0CW28VQJ4 |
Author | Floyd Bax |
Year | 2024 |
Publisher | FLOYD BAX; 1st edition |
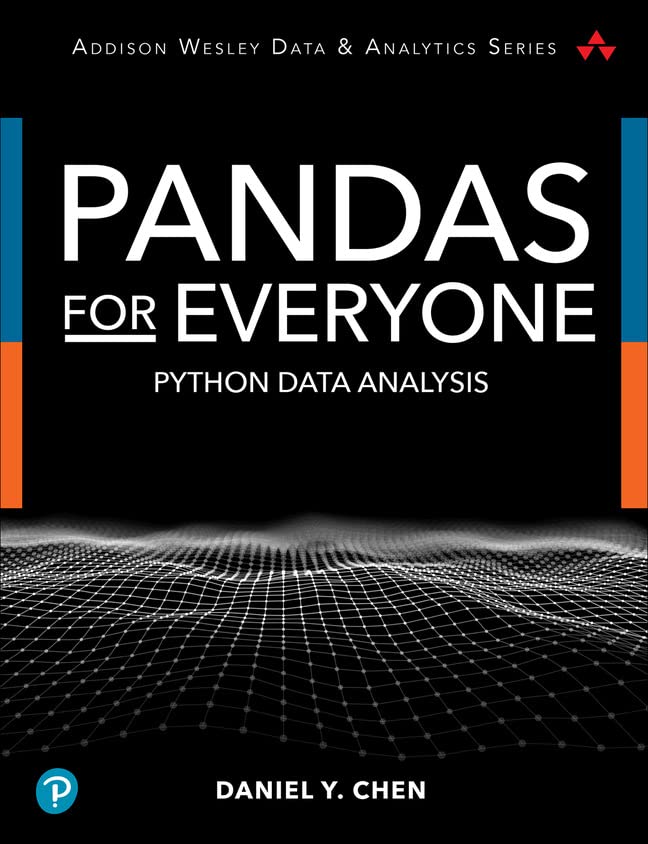 | Title | Pandas for Everyone: Python Data Analysis |
ISBN | 978-0-137-89115-3 |
Author | Daniel Chen |
Year | 2023 |
Publisher | Addison-Wesley Professional |
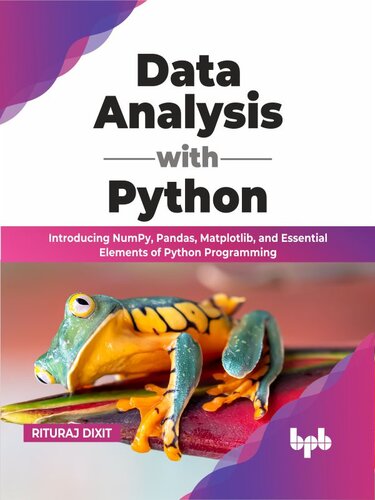 | Title | Data Analysis with Python: Introducing NumPy, Pandas, Matplotlib, and Essential Elements of Python Programming |
ISBN | 978-9-355-51065-5 |
Author | Rituraj Dixit |
Year | 2023 |
Publisher | BPB Publications |
.jpg) | Title | Python for Data Analysis: Data Wrangling with pandas, NumPy, and Jupyter |
ISBN | 978-1-098-10403-0 |
Author | Wes McKinney |
Year | 2022 |
Publisher | O'Reilly Media |
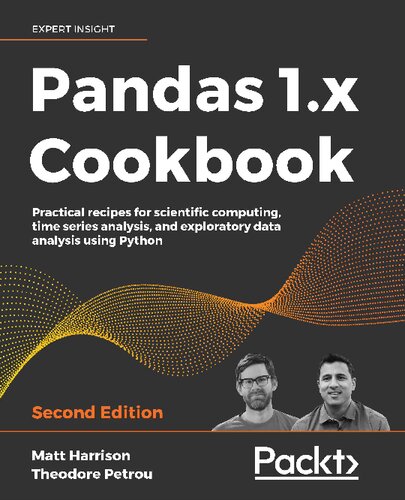 | Title | Pandas 1.x Cookbook: Practical recipes for scientific computing, time series analysis, and exploratory data analysis using Python |
ISBN | 978-1-839-21310-6 |
Author | Matt Harrison, Theodore Petrou |
Year | 2020 |
Publisher | Packt Publishing |
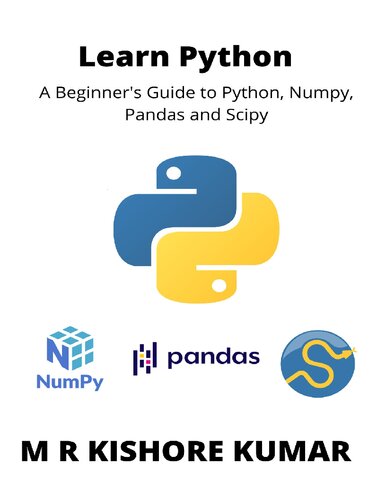 | Title | Learn Python: A Beginner's Guide to Python, Numpy,Pandas and Scipy |
ISBN | B098YPMHDB |
Author | Kumar, Kishore |
Year | 2021 |
Publisher | |
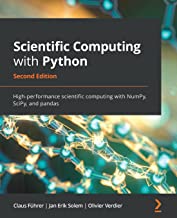 | Title | Scientific Computing with Python: High-performance scientific computing with NumPy, SciPy, and pandas |
ISBN | 978-1-838-82510-2 |
Author | Claus Fuhrer; Jan Erik Solem; Olivier Verdier |
Year | 2021 |
Publisher | Packt Publishing Ltd |
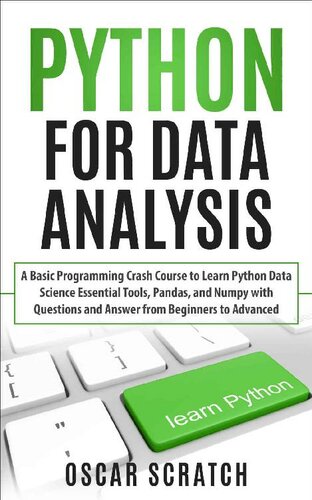 | Title | Python for Data Analysis: A Basic Programming Crash Course to Learn Python Data Science Essential Tools, Pandas, and Numpy with Questions and Answer from Beginners to Advanced |
ISBN | B07Z6H5Y7H |
Author | Oscar Scratch |
Year | 2019 |
Publisher | |
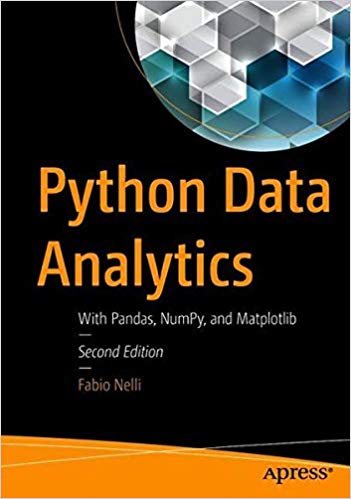 | Title | Python Data Analytics: With Pandas, NumPy, and Matplotlib |
ISBN | 978-1-484-23912-4 |
Author | Fabio Nelli |
Year | 2018 |
Publisher | Apress |
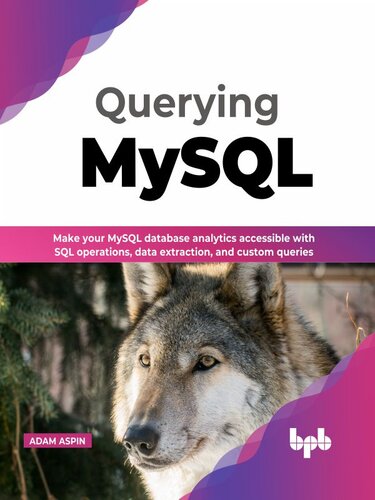 | Title | Querying MySQL |
ISBN | 978-9-355-51267-3 |
Author | Aspin, Adam |
Year | 2022 |
Publisher | BPB Publications |
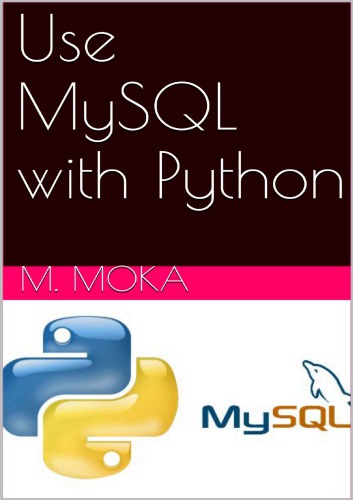 | Title | Use MySQL with Python |
ISBN | |
Author | M. MOka |
Year | 2016 |
Publisher | |
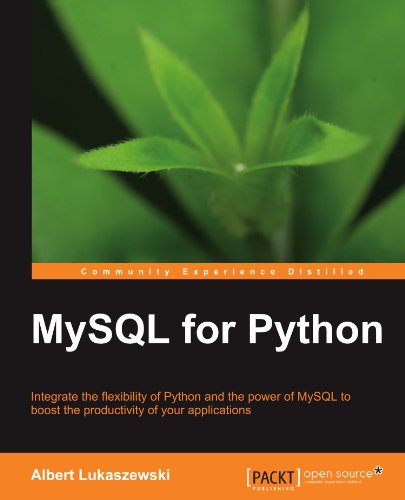 | Title | MySQL for Python |
ISBN | 978-1-849-51018-9 |
Author | Albert Lukaszewski |
Year | 2010 |
Publisher | Packt Publishing |
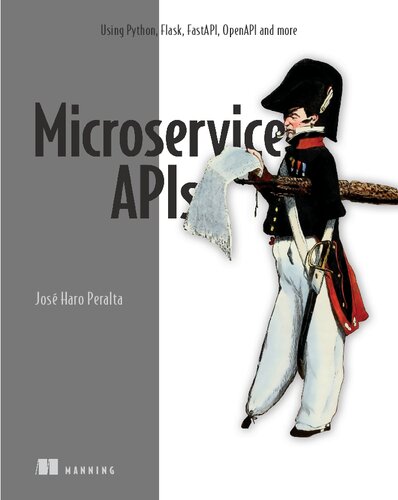 | Title | Microservice APIs: Using Python, Flask, FastAPI, OpenAPI and more |
ISBN | 978-1-617-29841-7 |
Author | José Haro Peralta |
Year | 2023 |
Publisher | Manning Publications |
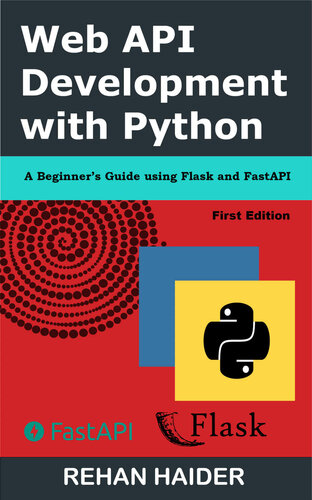 | Title | Web API Development with Python: A Beginner's Guide using Flask and FastAPI |
ISBN | B09BJLKM6F |
Author | Rehan Haider |
Year | 2021 |
Publisher | |
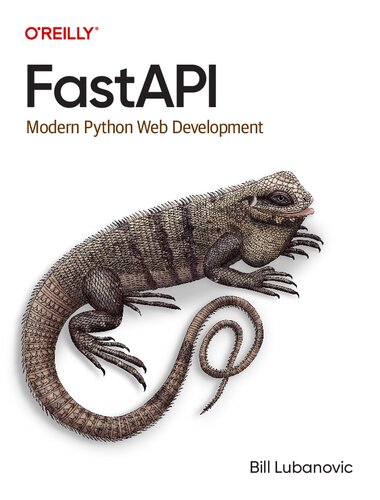 | Title | FastAPI: Modern Python Web Development |
ISBN | 978-1-098-13550-8 |
Author | Bill Lubanovic |
Year | 2023 |
Publisher | O'Reilly Media |
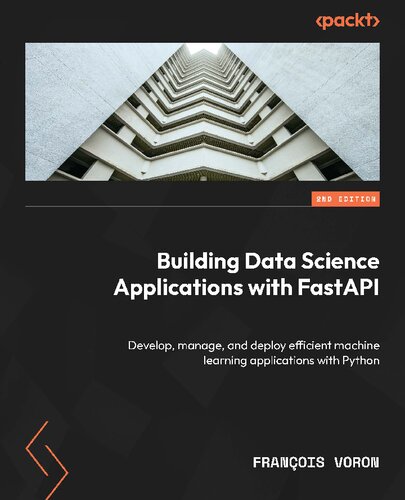 | Title | Building Data Science Applications with FastAPI: Develop, manage, and deploy efficient machine learning applications with Python |
ISBN | 978-1-837-63274-9 |
Author | Francois Voron |
Year | 2023 |
Publisher | Packt Publishing |
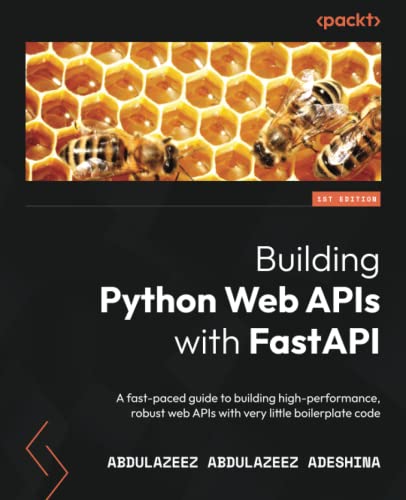 | Title | Building Python Web APIs with FastAPI: A fast-paced guide to building high-performance, robust web APIs with very little boilerplate code |
ISBN | 978-1-801-07663-0 |
Author | Abdulazeez Abdulazeez Adeshina |
Year | 2022 |
Publisher | Packt Publishing |
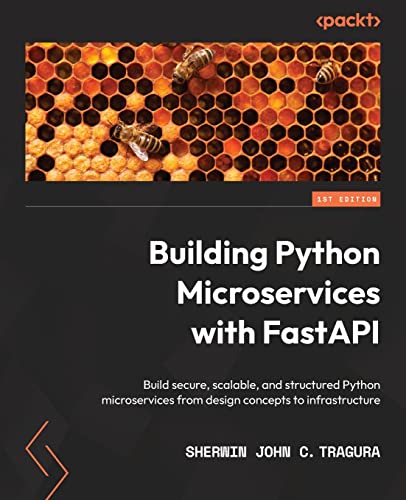 | Title | Building Python Microservices with FastAPI: Build secure, scalable, and structured Python microservices from design concepts to infrastructure |
ISBN | 978-1-803-24596-6 |
Author | Sherwin John Tragura |
Year | 2022 |
Publisher | Packt Publishing |